Hello friends, welcome to our new tutorial, in this android tutorial we will create a simple Android Upload Image to Server App using PHP and MySQL.
Android Upload Image using Android Upload Service
In this tutorial we will upload small image files from android to server. If need to upload larger files like a captured video check this tutorial
Android Upload Video to Server using PHP
If you want to see what exactly we will be creating in this Android Upload Image to Server app then you can see this video demonstration first.
Android Upload Image to Server Video Demonstration
If you liked the demonstration of Android Upload Image to Server App then go ahead to know how you can do this. Lets start creating our Android Upload Image to Server Project.
This Android Upload Image to Server is having two parts
- PHP MySQL
- Android Studio
Lets start with PHP MySQL for your Android Upload Image to Server app.
Android Upload Image to Server – PHP MySQL Part
- I am using hostinger hosting as my web server.
- So go to your hosting accounts PhpMyAdmin and create a new table.
- I have created the following table

- Now we need to create a php script for our database connection
- Create a new file named dbConnect.php and write the following code
1 2 3 4 5 6 7 8 9 |
<?php define('HOST','mysql.hostinger.in'); define('USER','u502452270_andro'); define('PASS','belal_123'); define('DB','u502452270_andro'); $con = mysqli_connect(HOST,USER,PASS,DB) or die('Unable to Connect'); |
- Now we need one more php script to get and save our image sent from android device
- Create a new file upload.php and write the following code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
<?php if($_SERVER['REQUEST_METHOD']=='POST'){ $image = $_POST['image']; require_once('dbConnect.php'); $sql = "INSERT INTO images (image) VALUES (?)"; $stmt = mysqli_prepare($con,$sql); mysqli_stmt_bind_param($stmt,"s",$image); mysqli_stmt_execute($stmt); $check = mysqli_stmt_affected_rows($stmt); if($check == 1){ echo "Image Uploaded Successfully"; }else{ echo "Error Uploading Image"; } mysqli_close($con); }else{ echo "Error"; } |
- Now upload both the files to your hosting account
- We need the address of upload.php file
- In my case the address of my upload.php is -> http://simplifiedcoding.16mb.com/ImageUpload/upload.php
Android Upload Image to Server – The Android Part
- Open android studio and create a new android project. (I have created ImageUploadSample)
- Firstly we will create a new class to handle our Http Request
- Right click on your package name and create a new java class (I created RequestHandler.java)
- Write the following code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 |
package net.simplifiedcoding.imageuploadsample; import java.io.BufferedReader; import java.io.BufferedWriter; import java.io.InputStreamReader; import java.io.OutputStream; import java.io.OutputStreamWriter; import java.io.UnsupportedEncodingException; import java.net.HttpURLConnection; import java.net.URL; import java.net.URLEncoder; import java.util.HashMap; import java.util.Map; import javax.net.ssl.HttpsURLConnection; /** * Created by Belal on 8/19/2015. */ public class RequestHandler { public String sendGetRequest(String uri) { try { URL url = new URL(uri); HttpURLConnection con = (HttpURLConnection) url.openConnection(); BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(con.getInputStream())); String result; StringBuilder sb = new StringBuilder(); while((result = bufferedReader.readLine())!=null){ sb.append(result); } return sb.toString(); } catch (Exception e) { return null; } } public String sendPostRequest(String requestURL, HashMap<String, String> postDataParams) { URL url; String response = ""; try { url = new URL(requestURL); HttpURLConnection conn = (HttpURLConnection) url.openConnection(); conn.setReadTimeout(15000); conn.setConnectTimeout(15000); conn.setRequestMethod("POST"); conn.setDoInput(true); conn.setDoOutput(true); OutputStream os = conn.getOutputStream(); BufferedWriter writer = new BufferedWriter( new OutputStreamWriter(os, "UTF-8")); writer.write(getPostDataString(postDataParams)); writer.flush(); writer.close(); os.close(); int responseCode = conn.getResponseCode(); if (responseCode == HttpsURLConnection.HTTP_OK) { BufferedReader br = new BufferedReader(new InputStreamReader(conn.getInputStream())); response = br.readLine(); } else { response = "Error Registering"; } } catch (Exception e) { e.printStackTrace(); } return response; } private String getPostDataString(HashMap<String, String> params) throws UnsupportedEncodingException { StringBuilder result = new StringBuilder(); boolean first = true; for (Map.Entry<String, String> entry : params.entrySet()) { if (first) first = false; else result.append("&"); result.append(URLEncoder.encode(entry.getKey(), "UTF-8")); result.append("="); result.append(URLEncoder.encode(entry.getValue(), "UTF-8")); } return result.toString(); } } |
- In activity_main.xml we have to create the following layout
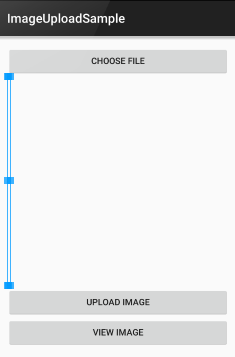
- For creating the above layout you can use the following xml code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:orientation="vertical" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity"> <Button android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="@string/string_choose_file" android:id="@+id/buttonChoose" /> <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="1" android:id="@+id/imageView" /> <Button android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="@string/string_upload_image" android:id="@+id/buttonUpload" /> <Button android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="@string/string_view_image" android:id="@+id/buttonViewImage" /> </LinearLayout> |
- Now come to MainActivity.java file
- First we will declare some variables
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
public static final String UPLOAD_URL = "http://simplifiedcoding.16mb.com/ImageUpload/upload.php"; public static final String UPLOAD_KEY = "image"; public static final String TAG = "MY MESSAGE"; private int PICK_IMAGE_REQUEST = 1; private Button buttonChoose; private Button buttonUpload; private ImageView imageView; private Bitmap bitmap; private Uri filePath; |
- Inside onCreate method we will initialize our components
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); buttonChoose = (Button) findViewById(R.id.buttonChoose); buttonUpload = (Button) findViewById(R.id.buttonUpload); buttonView = (Button) findViewById(R.id.buttonViewImage); imageView = (ImageView) findViewById(R.id.imageView); buttonChoose.setOnClickListener(this); buttonUpload.setOnClickListener(this); } |
- As you can see we have added setOnClickListener to the buttons.
- So now come to your onClick method and write the following code
1 2 3 4 5 6 7 8 9 10 11 |
@Override public void onClick(View v) { if (v == buttonChoose) { showFileChooser(); } if(v == buttonUpload){ uploadImage(); } } |
- Here we have two methods showFileChooser() this method will show the gallery from where user can choose image to upload.
- When the user will choose an Image to upload, then after selection we will show that image to the ImageView we created in our main layout.
- These two methods will do all the require things
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
private void showFileChooser() { Intent intent = new Intent(); intent.setType("image/*"); intent.setAction(Intent.ACTION_GET_CONTENT); startActivityForResult(Intent.createChooser(intent, "Select Picture"), PICK_IMAGE_REQUEST); } @Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { super.onActivityResult(requestCode, resultCode, data); if (requestCode == PICK_IMAGE_REQUEST && resultCode == RESULT_OK && data != null && data.getData() != null) { filePath = data.getData(); try { bitmap = MediaStore.Images.Media.getBitmap(getContentResolver(), filePath); imageView.setImageBitmap(bitmap); } catch (IOException e) { e.printStackTrace(); } } } |
- Now we have the Image which is to bet uploaded in bitmap.
- We will convert this bitmap to base64 string
- So we will create a method to convert this bitmap to base64 string
1 2 3 4 5 6 7 8 9 |
public String getStringImage(Bitmap bmp){ ByteArrayOutputStream baos = new ByteArrayOutputStream(); bmp.compress(Bitmap.CompressFormat.JPEG, 100, baos); byte[] imageBytes = baos.toByteArray(); String encodedImage = Base64.encodeToString(imageBytes, Base64.DEFAULT); return encodedImage; } |
- Now finally create uploadImage() method
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
private void uploadImage(){ class UploadImage extends AsyncTask<Bitmap,Void,String>{ ProgressDialog loading; RequestHandler rh = new RequestHandler(); @Override protected void onPreExecute() { super.onPreExecute(); loading = ProgressDialog.show(MainActivity.this, "Uploading Image", "Please wait...",true,true); } @Override protected void onPostExecute(String s) { super.onPostExecute(s); loading.dismiss(); Toast.makeText(getApplicationContext(),s,Toast.LENGTH_LONG).show(); } @Override protected String doInBackground(Bitmap... params) { Bitmap bitmap = params[0]; String uploadImage = getStringImage(bitmap); HashMap<String,String> data = new HashMap<>(); data.put(UPLOAD_KEY, uploadImage); String result = rh.sendPostRequest(UPLOAD_URL,data); return result; } } |
- Now thats it for Android Image Upload to Server. You can see the final complete code below for MainActivity.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 |
package net.simplifiedcoding.imageuploadsample; import android.app.ProgressDialog; import android.content.Context; import android.content.Intent; import android.database.Cursor; import android.graphics.Bitmap; import android.net.Uri; import android.os.AsyncTask; import android.provider.MediaStore; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.util.Base64; import android.util.Log; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.Button; import android.widget.ImageView; import android.widget.Toast; import java.io.ByteArrayOutputStream; import java.io.IOException; import java.util.HashMap; public class MainActivity extends AppCompatActivity implements View.OnClickListener { public static final String UPLOAD_URL = "http://simplifiedcoding.16mb.com/ImageUpload/upload.php"; public static final String UPLOAD_KEY = "image"; public static final String TAG = "MY MESSAGE"; private int PICK_IMAGE_REQUEST = 1; private Button buttonChoose; private Button buttonUpload; private ImageView imageView; private Bitmap bitmap; private Uri filePath; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); buttonChoose = (Button) findViewById(R.id.buttonChoose); buttonUpload = (Button) findViewById(R.id.buttonUpload); buttonView = (Button) findViewById(R.id.buttonViewImage); imageView = (ImageView) findViewById(R.id.imageView); buttonChoose.setOnClickListener(this); buttonUpload.setOnClickListener(this); } private void showFileChooser() { Intent intent = new Intent(); intent.setType("image/*"); intent.setAction(Intent.ACTION_GET_CONTENT); startActivityForResult(Intent.createChooser(intent, "Select Picture"), PICK_IMAGE_REQUEST); } @Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { super.onActivityResult(requestCode, resultCode, data); if (requestCode == PICK_IMAGE_REQUEST && resultCode == RESULT_OK && data != null && data.getData() != null) { filePath = data.getData(); try { bitmap = MediaStore.Images.Media.getBitmap(getContentResolver(), filePath); imageView.setImageBitmap(bitmap); } catch (IOException e) { e.printStackTrace(); } } } public String getStringImage(Bitmap bmp){ ByteArrayOutputStream baos = new ByteArrayOutputStream(); bmp.compress(Bitmap.CompressFormat.JPEG, 100, baos); byte[] imageBytes = baos.toByteArray(); String encodedImage = Base64.encodeToString(imageBytes, Base64.DEFAULT); return encodedImage; } private void uploadImage(){ class UploadImage extends AsyncTask<Bitmap,Void,String>{ ProgressDialog loading; RequestHandler rh = new RequestHandler(); @Override protected void onPreExecute() { super.onPreExecute(); loading = ProgressDialog.show(MainActivity.this, "Uploading Image", "Please wait...",true,true); } @Override protected void onPostExecute(String s) { super.onPostExecute(s); loading.dismiss(); Toast.makeText(getApplicationContext(),s,Toast.LENGTH_LONG).show(); } @Override protected String doInBackground(Bitmap... params) { Bitmap bitmap = params[0]; String uploadImage = getStringImage(bitmap); HashMap<String,String> data = new HashMap<>(); data.put(UPLOAD_KEY, uploadImage); String result = rh.sendPostRequest(UPLOAD_URL,data); return result; } } UploadImage ui = new UploadImage(); ui.execute(bitmap); } @Override public void onClick(View v) { if (v == buttonChoose) { showFileChooser(); } if(v == buttonUpload){ uploadImage(); } } } |
So thats it for our Android Upload Image to Server app. In the next part of Android Upload Image to Server app we will see how we can fetch the uploaded images. You can also download the source code of this Android Upload Image to Server app from the link given below.
Android Upload Image to Server – Download Source Code
[wp_ad_camp_1]
[sociallocker id=”1372″] [download id=”1395″] [/sociallocker]
Next Part – Download Image from Server using PHP MySQL.